|
SESSION 6 Part4 |
Source File Structure |
|
|
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
Okay,
now that the ritual is complete,
let's take a basic look at
the
structure of the source file. |
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
 |
 |
 |
|
|
|
|
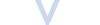 |
|
|
|
|
|
|
|
|
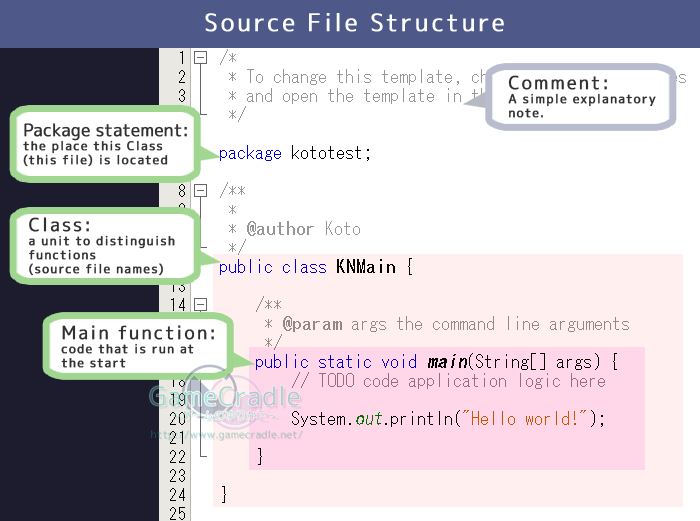
|
|
|
 |
|
 |
|
Your first program code(KotoTest's KNMain.java)
|
|
001 |
|
/* |
<- |
comment using /**/ |
002 |
|
*
To change this template, choose Tools | Templates |
|
|
003 |
|
*
and open the template in the editor. |
|
|
004 |
|
*/ |
|
|
005 |
|
|
|
|
006 |
|
package kototest; |
|
|
007 |
|
|
|
|
008 |
|
/** |
<- |
comment using /**/ |
009 |
|
* |
|
|
010 |
|
*
@author Koto |
|
|
011 |
|
*/ |
|
|
012 |
|
public
class KNMain { |
|
|
013 |
|
|
|
|
014 |
|
/** |
<- |
comment using /**/ |
015 |
|
* @param args the command line arguments |
|
|
016 |
|
*/ |
|
|
017 |
|
public static void
main(String[] args) { |
|
|
018 |
|
// TODO code application logic here |
<- |
comment using // |
019 |
|
|
|
|
020 |
|
System.out.println("Hello
world!"); |
|
|
021 |
|
|
|
|
022 |
|
} |
|
|
023 |
|
|
|
|
024 |
|
} |
|
|
|
|
|
|
 |
|
 |
|
|
|
|
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
First we'll start with comments.
These yellow parts (with grey text)
are comments.
A comment is a specific
explanatory note
for that item.
It is not a program.
Look, even if you make a program,
when you look at it later, you might
forget what you were doing, right?
If you write memos and explanations
within your program, then you'll have
some security. |
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
Really, so you can write
memos inside your code!
That'll really help me. |
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
|
|
|
|
|
|
|
 |
|
 |
|
How to write a comment |
|
Method |
Usage |
// |
The characters after "//" will become a
comment.
This can only be used to turn a single line
into a
comment. |
/*
*/ |
Enclose the part that you want to turn into a
comment
with "/*" and "*/".
"/*" is the start, and "*/" is the end.
You can turn multiple lines into a comment. |
|
|
|
Examples |
// |
//++++++++++++++++++++++++++++
// Koto is an idiot.
// As a matter of fact, she's not sexy all.
// She also has no brains.
//++++++++++++++++++++++++++++ |
/*
*/ |
/* NAPO is a total genius.
*/
/* Oh yeah. He's a good-looking guy,
the most wonderful in the world. */
/*
+ By the way, that Koto chick
+ is completely hopeless. Just kidding.
*/ |
|
|
|
|
|
|
 |
|
 |
|
Hoho
Hoho |
|
|
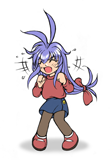 |
What the heck
is this!! |
|
|
|
|
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
With Java, there's two ways for writing comments.
These methods use "//", or "/*" and "*/".
Each has their own place to be used, so make sure to use
them
properly.
The parts that have been made into comments
won't be viewed as
executable program by the
Java compiler. They'll be ignored.
So, you can write comments anywhere in the
source file.
In other words, no matter where you write these
sentence,
they won't create errors.
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
If they annoy you that much,
practice writing your own
comments. |
|
|
 |
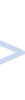 |
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
|
|
|
|
|
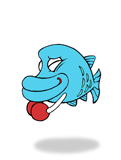 |
Can you actually
write a comment? |
|
I won't show
you, though. |
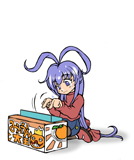 |
|
|
|
What do you mean!
Show me!
I mean, not that I
really care that much.
I bet you couldn't
even do it.
|
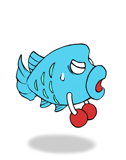 |
|
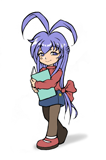
|
I did it!
And it definitely
went grey.
So then I deleted it~
What a shame, huh! |
|
|
|
|
|
|
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
Hmmm…
Okay, so for now, is everything
fine other than the Main function?
For now we'll skip the other
entries in the picture.
It's fine if you just glance over the
contents
of the picture.
Just leave them alone,
and don't rewrite them.
There's nothing immediately relevant
besides the Main function,
and this is closely related to classes,
so I'll explain them all together later.
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
 |
 |
 |
|
|
|
|
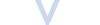 |
|
|
|
|
|
|
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
There's, like,
all sorts of
classes
there, huh.
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
 |
 |
 |
|
|
|
|
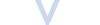 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
In Java programming,
classes are important components
that affect the core.
But that part is a huge explanation
that's difficult to understand.
So let's go slowly and not get flustered. |
|
|
 |
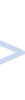 |
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
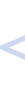 |
 |
|
Okay!
Slow and
steady wins the
race, right! |
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
|
|
|
|
|
|
|
|
The flow of program run-time |
|
|
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
Next, let's take a look at the
flow of
program run-time for a
Java application,
using this program.
At the same time let's look at the
Main function.
The flow of the program is something
like this. |
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
 |
 |
 |
|
|
|
|
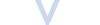 |
|
|
|
|
|
|
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
Ooh,
so it's this sort
of flow.
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
 |
 |
 |
|
|
|
|
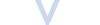 |
|
|
|
|
|
|
|
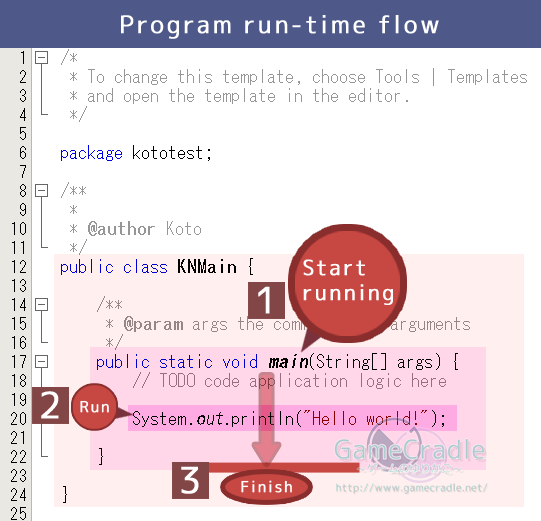
|
 |
|
 |
|
|
Run-time flow |
1 |
The Java application runs.
First the main function is invoked,
and execution of the processes written within it begin. |
2 |
The contents of the Main function block are run from the
top in order.
They are run one at a time, moving to the next one as
each finishes.
This time, the process that was run was to display
"Hello world!" in the standard output. |
3 |
When the processing is completed at the end of the
block, the program finishes.
|
|
|
|
|
|
 |
|
 |
|
|
Simple,
right? |
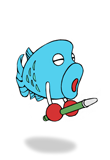 |
|
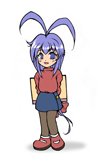 |
So it ends when
gets to the bottom… |
|
|
|
|
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
This time, because there's only one command,
which is to
display a message in the standard output,
it finishes once
that's been run.
If there were multiple commands,
they would run in order
from the top…
going to the next one as each finishes.
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
|
|
|
|
|
|
|
Main function (Main method) |
|
|
|
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
This time, we wrote
the
program in a section called the
main function (main method).
This is a special process that is
invoked at the beginning
when a
Java application is run.
This is the starting point where
the run process
of the application
starts.
You can only make one of these
in each Java application. |
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
 |
 |
 |
|
|
|
|
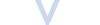 |
|
|
|
|
|
|
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
So it's special.
You write the program
inside it, right?
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
 |
 |
 |
|
|
|
|
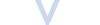 |
|
|
|
|
To put it another way
it's something
that's always required.
|
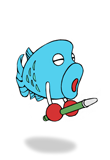 |
|
|
That reminds me,
I saw it last time.
|
|
|
 |
|
 |
|
Features of the Main function
|
* Only one can be written in each Java application. |
* It is invoked at the start of the run-time. |
|
|
|
|
 |
|
 |
|
|
 |
|
 |
|
Your first program code(KotoTest's KNMain.java) |
|
001 |
|
/* |
|
|
002 |
|
* To
change this template, choose Tools | Templates |
|
|
003 |
|
* and
open the template in the editor. |
|
|
004 |
|
*/ |
|
|
005 |
|
|
|
|
006 |
|
package kototest; |
|
|
007 |
|
|
|
|
008 |
|
/** |
|
|
009 |
|
* |
|
|
010 |
|
*
@author Koto |
|
|
011 |
|
*/ |
|
|
012 |
|
public class
KNMain { |
|
|
013 |
|
|
|
|
014 |
|
/** |
|
|
015 |
|
*
@param args the command line arguments |
|
|
016 |
|
*/ |
|
|
017 |
|
public static void
main(String[] args) { |
<- |
Main Function |
018 |
|
// TODO code
application logic here |
|
|
019 |
|
|
|
|
020 |
|
System.out.println("Hello
world!"); |
|
|
021 |
|
|
|
|
022 |
|
} |
|
|
023 |
|
|
|
|
024 |
|
} |
|
|
|
|
|
|
|
 |
|
 |
|
|
|
|
|
|
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
Ah yes, the program is written
between
the
brackets
"{" and
"}" of the
main function.
The range for these brackets is
called a
'block'.
Be careful of the different brackets.
The brackets for the declaration
part
are ( and
).
The brackets for the block are { and
}.
You can't use [ and ].
A lot of different brackets are
used
with Java,
but each has a different
purpose.
So be careful.
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
Blocks have to be
closed at the start
and finish
using
brackets.
The start of a
block is
"{"
and the end of a
block is
"}".
|
|
|
|
|
|
 |
 |
|
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|